In this post, we will learn to pull the spreadsheet list available into our Google Doc account through .NET. I created the following Form into MS-Visual Studio. The form has a button and a list view control. We will write the .NET code logic on button click event to pull the list of spreadsheets from Google Doc
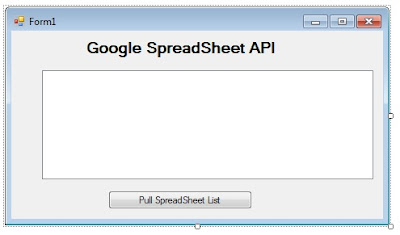
I am using following Google Data API namespaces on this form.
using Google.GData; using Google.GData.Client; using Google.GData.Extensions; using Google.GData.Spreadsheets;
I have written following .NET code snippet on button "Pull SpreadSheet List"
private void button1_Click(object sender, EventArgs e) { listView1.Columns.Add("SpreadSheet Name"); listView1.Columns.Add("Summary"); listView1.Columns.Add("Published Date"); SpreadsheetsService GoogleExcelService; GoogleExcelService = new SpreadsheetsService("Spreadsheet-Vikash-Test-App"); GoogleExcelService.setUserCredentials("abc@gmail.com", "abcpassword"); SpreadsheetQuery query = new SpreadsheetQuery(); SpreadsheetFeed myFeed = GoogleExcelService.Query(query); foreach (SpreadsheetEntry mySpread in myFeed.Entries) { listView1.Items.Add(new ListViewItem(new string[] { mySpread.Title.Text, mySpread.Summary.Text, mySpread.Updated.ToShortDateString() })); }
If you observe the code it is very similar to what we did into Picasa Web Album API series.
We have created a new Spreadsheet Service and pass on the user credentials i.e. the Google Doc account information. This is needed since we are using Client Login Authentication method to access Google online services. There are other authentication methods available to connect with Google online services (OAuth 2.0 and OAuth 1.0)
We have created a query and pass it to Google Spreadsheet services which in turn return a feed. This feed contains entries and each entry represents a Spreadsheet. I looped through the foreach loop and read each entry. Since each entry is a spreadsheet; we can read their properties (Title, Summary etc.).
I connected with to the internet and run the form. I clicked on button Pull SpreadSheet List and it pulled the list of Spreadsheet available into my Google Doc account.
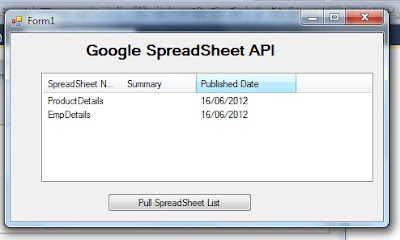
The next thing I did was to navigate to the Google Doc and checked what files are available in my account. The two sample files were smiling over there.
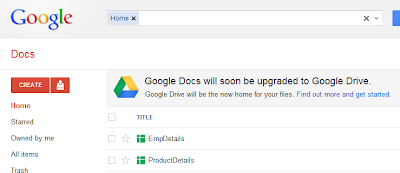
So we have learn to pull list of all SpreadSheet from Google Doc using SpreadSheet API.
Thanks for reading this post